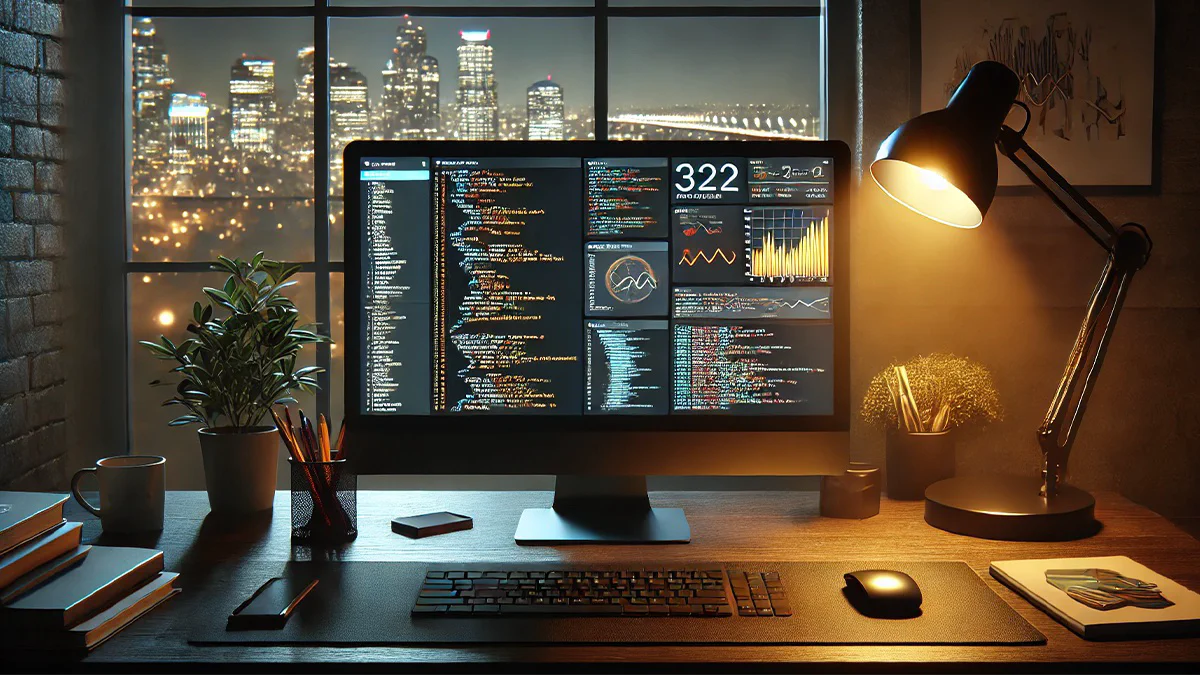
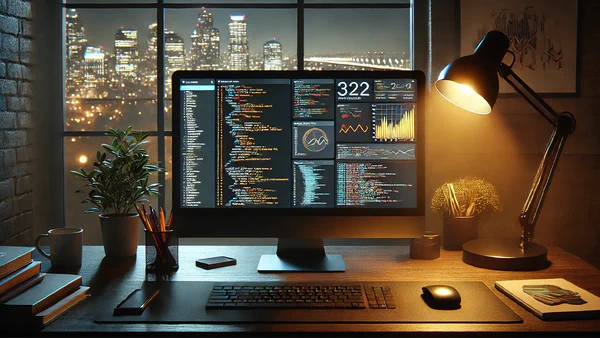
1. What is the <Transition> component?
Vue.js offers us the <Transition> component, which allows us to achieve the effect of smooth transitions between component states. It makes it easier to create enter and leave animations for displayed application elements. The <Transition> component is based on specific points such as: "before-enter", "enter", "after-enter". We can also define these points for the leave state - "before-leave", "leave", "after-leave". By defining them appropriately, we can control various properties of the transition, e.g. duration, delay, softening functions, etc. The use of smooth transitions and animations has a positive impact on the interactive UI and ensures a positive user experience.
2. How to use the <Transition> component
The <Transition> component is a pre-existing component, meaning it's readily accessible in any component's template without requiring explicit registration. Transitions are typically triggered by conditional display via v-show directive or conditional rendering via v-if directive.
|
|
3. Transition CSS classes
The
v-enter-from: Added before the start of the enter transition.
v-enter-active: Added during the enter transition.
v-enter-to: Added after the enter transition has finished.
v-leave-from: Added before the start of the leave transition.
v-leave-active: Added during the leave transition.
v-leave-to: Added after the leave transition has finished.
When we provide a name through the "name" parameter, it will be used as a prefix for the CSS classes, allowing for personalized class names according to the project's needs. For example, if we set the "name" parameter to the value "button", the CSS classes related to the transition will have names such as "button-enter", " button-enter-active", "button-enter-to", "button-leave", "button-leave-active", "button-leave-to". This enables us to easily identify classes associated with a particular transition and customize their styling according to our preferences.
|
|
4. Transition modes
The <Transition> component can also specify the order in which elements are inserted and removed. This is possible through the mode parameter, whose default value is "in-out". In this case, the entering animation begins only after the leaving animation has finished. The second mode is "out-in", which means, the leave transition after the enter transition completes.
|
|
5. Sample implementation of Vue <Transition> component
Here's a sample implementation of switching between dark and light mode using the Vue <Transition> component:
|
|
In the above example, we have a button that toggles between dark and light modes upon clicking. The buttons are wrapped inside a <Transition> component, which change mode value when switching between modes.
name="button":This sets the name of the transition. It's used to define the CSS classes for the transition.
mode="out-in":This sets the mode of the transition. The "out-in" mode first triggers the leave transition on the current element, then triggers the enter transition on the new element. It ensures smooth transition effects when elements are replaced.
Improve your UX/UI today
Creating smooth transitions between states using the <Transition> component is very simple and produces brilliant results. It significantly improves the positive user experience and, consequently, the attractiveness of the application. In addition, Vue.js also offers <TransitionGroup> which can be used to create transitions for a list of elements. Improve your UX/UI today and drop us a line here.
Written by:
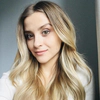
Ewelina Zwolenik
Frontend Developer
Ewelina is an amazing frontend developer who's great at working with Vue.js, HTML, CSS, and Tailwind CSS. She has a sharp eye for design and knows how to turn ideas into beautiful, responsive websites and apps that look great on any device.
Read more like this:
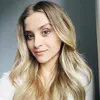
Ewelina Zwolenik
How to Test VueRouter With Vitest
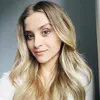
Ewelina Zwolenik
CSS Flexbox vs CSS Grid explained
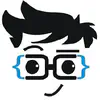
Chandra Sekar
Vue JS make developer life easier
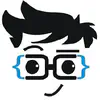
Chandra Sekar
How to Effectively Mock API Calls using Vue.js and Axios
Got idea?Let’s talk about your project
Schedule a call with us