Difficulty Level: Intermediate
Prerequisite: You should be familiar with Vue.js application and Axios as HTTP client in your project
Takeaways: This article helps to understand a practical approach to mocking api requests in frontend


Understanding the need for API mocking:
APIs play a pivotal role in modern web development, connecting frontend applications with backend services. However, relying on actual APIs during development and testing can introduce challenges, such as dependencies on external services, unpredictable responses, and potential rate limiting. Mocking API calls becomes imperative to create a controlled and predictable environment for frontend development.
Installation and Project Setup
Install and Setup Vue app
To initiate a Vue project, ensure you have Node.js installed. Open a terminal and run the below command
|
|
This installs the Vue CLI globally. Next, create a new project with
|
|
Navigate to the project folder using cd project-name. To serve the project locally, run
|
|
This deploys a development server, and you can view the project at http://localhost:8080 in your browser. Customize your Vue project further by exploring the src directory.
Install Axios Library
To install Axios in vue project, a popular HTTP client for JavaScript
|
|
Simple Axios configuration to fetch data from backend
|
|
What are Interceptors in Axios?
In Axios, interceptors are functions that allow developers to intercept and manipulate HTTP requests and responses globally before they are sent or after they are received. These interceptors provide a powerful mechanism to customize, modify, or handle aspects of the request or response flow. Axios supports both request and response interceptors, offering flexibility in managing API communication.
Configuring Axios Instance with interceptors
Let's take a simple example of Vue application and configure axios to mock api calls
Project folder structure:
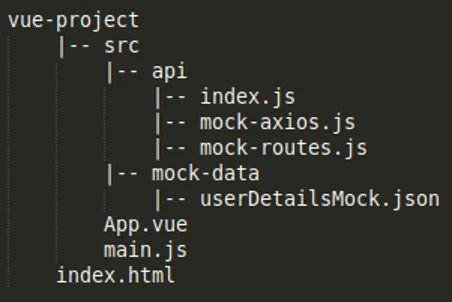
Write Code to mock Api calls with Axios
Filename -> vue-project/src/App.vue
|
|
Filename -> vue-project/src/api/index.js
|
|
Filename -> vue-project/src/api/mock-axios.js
|
|
Filename -> vue-project/src/api/mock-routes.js
|
|
Filename -> vue-project/src/mock-data/userDetailsMock.json
|
|
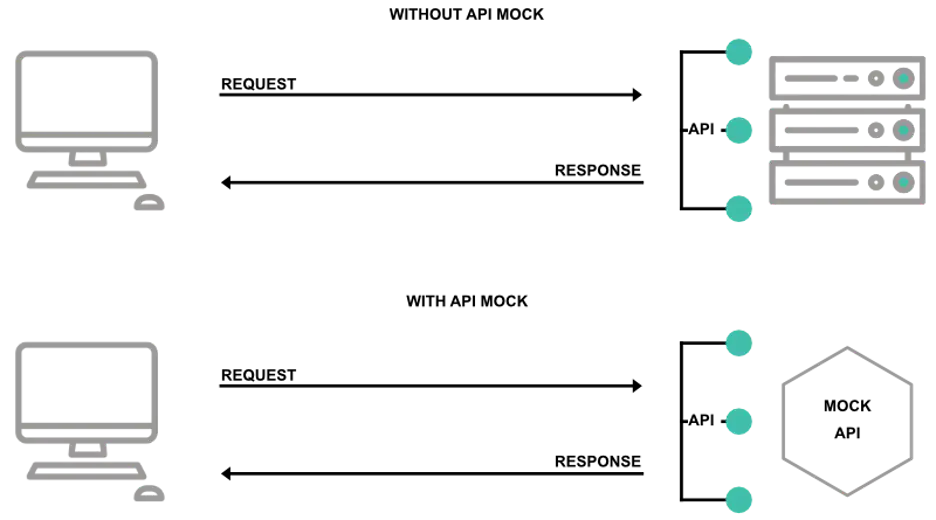
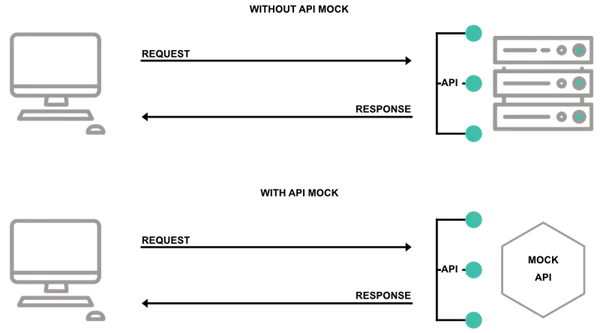
Conclusion
Axios interceptors are a powerful tool that empowers developers to take control of their HTTP requests and responses. By leveraging interceptors, you can implement global configurations, handle errors consistently, implement caching layer, mock api calls and address various real-world use cases seamlessly.
If you're looking to optimize your Vue.js applications with expert techniques like Axios interceptors and mocking, our team at Meant4 is here to help. With our deep expertise in web development and API integration, we can elevate your projects and deliver tailored solutions. Contact us today to learn how we can support your development needs!
Written by:
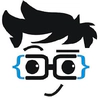
Chandra Sekar
Senior Frontend Developer
Chandra is an exceptional Vue.js frontend developer who consistently delivers outstanding results. With a keen eye for detail and a deep understanding of Vue.js, Chandra transforms design concepts into seamless and visually captivating user interfaces.
Read more like this:
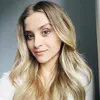
Ewelina Zwolenik
How to Test VueRouter With Vitest
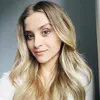
Ewelina Zwolenik
CSS Flexbox vs CSS Grid explained
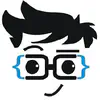
Chandra Sekar
How to add custom tags in Storybook
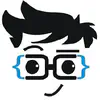
Chandra Sekar
Pinia.js over Vuex for Vue.js state management
Got idea?Let’s talk about your project
Schedule a call with us